Use and use of REST API communication in the AMAGE system
Introduction
The AMAGE system provides the ability to communicate with external systems using REST API. To facilitate integration with the AMAGE system, we provide sample scripts that can be used for integration with the AMAGE system. In this document, we present examples of using the REST API in the AMAGE system.
API keys, authorization and data headers
To enable access to the API and be able to communicate through this interface, we need to create API keys for authorizing/signing queries so that the AMAGE system is able to correctly recognize the query and share the data. Generate API keys in the configuration section and don’t forget to activate it. Save three parameters - device-uuid, key and secret, which will be used to generate the query signature.
More:
Object identification/UUID
Objects in the system identify themselves by their unique UUID. In order to download an object from the system, you need to know its UUID. UUID can be found in the user interface, in export files, in the database, in system logs. Typically, in the user interface, an additional button with an (I)
icon appears for each object in the data viewer or its editor. After selecting it, information about the object’s identification data, including UUID, is displayed. Use this UUID for interactions where a UUID is required.
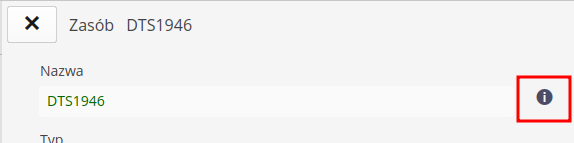
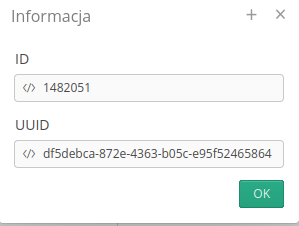
API signature
For each communication with the AMAGE system through the REST interface, three headers (headers) with data are required:
-
amage-device-uuid
- identifier of the device for which the signature is generated -
amage-api-key
- API key -
amage-api-sign
- query signature
Device UUID and API key are provided directly from the generated API key. The signature is generated based on the query path and the API key secret. The signature is generated using the SHA-256 algorithm and the result is transmitted in binary form. The result is then converted to hexadecimal and passed as an amage-api-sign
header.
More in the code examples below.
Example Downloading an attachment
To download an attachment from the AMAGE system, perform an HTTP GET request to the appropriate URL address. In the query, you must provide the UUID of the attachment you want to download. In response, we will receive a binary file that should be saved on the local disk.
Method: GET Path: rest/amage/v1/attachment/get/by-uuid/{uuid} Generates: APPLICATION_OCTET_STREAM_VALUE Requires: TEXT_PLAIN_VALUE, APPLICATION_JSON_VALUE Authorization: API signature (device-uuid, api-key , api-sign)
After executing the query, we will receive a binary file in response, which should be saved on the local disk. Python example code below.
Examples of downloading report content in JSON format
To receive the content of the report from the AMAGE system, perform an HTTP GET request to the appropriate URL address. In the query, you must provide the UUID of the tab of the report you want to download. In response, we will receive JSON data that can be processed in any way. In this case (for reports), we can send the device-uuid, api-key and directly api-secret parameters in the query. This makes it easier to generate queries and use API reports using Business Intelligence (BI) systems. It should be emphasized that the api-sign method always works.
Method: GET Path: rest/amage/v1/reports/generate/raw-json/by-bookmark-uuid/{uuid}
Example call via HTTP Client:
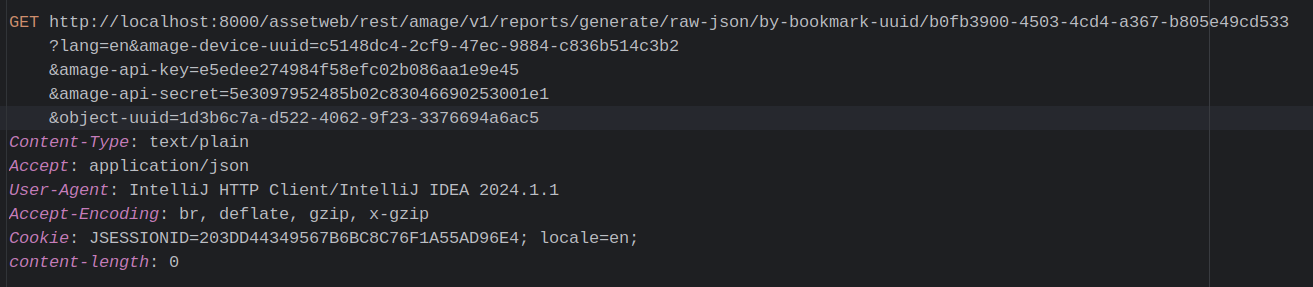
Query result for a JSON report:
{
"item": {
"GROUP_UUID": "",
"INVENTORY_NUMBER": "X/23/333/2023",
"TESTING_LABORATORY_UUID": "",
"MANUFACTURER_NAME": "AMAGE",
"TYPE_MANUFACTURER_UUID": "b133c9d3-a018-405e-b632-d124ea5c342e",
"SERIAL_NUMBER": "XX15/1/1[A]",
"MANUFACTURER_UUID": "b133c9d3-a018-405e-b632-d124ea5c342e",
"TYPE_MANUFACTURER_NAME": "AMAGE",
"MAP_SHAPE_MAP_NAME": "",
"NOTE": "Test device",
"PARENT_NAME": "100DE15 SPOOL-1",
"IS_EQUIPMENT": "false",
"UUID": "1d3b6c7a-d522-4062-9f23-3376694a6ac5",
"TESTING_LABORATORY_NAME": "",
"PARENT_UUID": "da6243db-abef-44ee-b535-3b1556de6407",
"IDENTIFIERS": " ",
"TYPE_CATALOG_NUMBER": "",
"LOCATION_UUID": "",
"NAME": "100DE15 SPOOL-1 PT-NO-1[A] IC 26_LL PIPE SL, CS, CE, TC2, 114.3X6.3",
"TYPE_UUID": "b4b0c37e-7c39-4946-ab81-7c373c17e37c",
"GROUP_NAME": "",
"TYPE_ORDER_NUMBER": "",
"EQUIPMENT_VALID_TILL_DATE": "-",
"MAP_SHAPE_LONGITUDE": "",
"MAP_SHAPE_LATITUDE": "",
"COUNT": "0,00",
"TYPE_NAME": "Type of the Asset",
"LOCATION_NAME": ""
}
}
Python example
The code in the example below shows a Python script that downloads an attachment from AMAGE. The script uses the requests
library to communicate with the server and hashlib
to generate the API signature. In place of <amage_server_address>
, enter the address of the AMAGE server.
#!/usr/bin/python3
import binascii
import requests
import hashlib
PARAM_SEPARATOR = ";"
path = 'attachment/get/by-uuid/d18ed480-febd-4f0c-a979-a773bca83423'
device_uuid = "86947df1-ba7d-46d7-83a8-a90e1fdca8bf"
api_key = "e3d1acd2c8b5e47d9e612cf46f08523f"
api_secret = "7fe1cde52dc14ecc4b222090c72b4380"
def calc_api_sign(path, api_secret):
message_digest = hashlib.sha256()
message_digest.update(api_secret)
message_digest.update(PARAM_SEPARATOR.encode('utf-8'))
message_digest.update(path.encode('utf-8'))
return message_digest.digest()
def download_file(url, headers, local_filename):
with requests.get(url, headers=headers, stream=True) as r:
r.raise_for_status()
with open(local_filename, 'wb') as f:
for chunk in r.iter_content(chunk_size=8192):
f.write(chunk)
return local_filename
api_sign = binascii.hexlify(calc_api_sign(path, bytearray.fromhex(api_secret))).decode()
url = "https://<amage_server_address>/rest/amage/v1/" + path
headers = {
"Content-Type": "text/plain",
"Accept": "application/octet-stream",
"amage-device-uuid": device_uuid,
"amage-api-key": api_key,
"amage-api-sign": api_sign
}
local_filename = "item.png"
download_file(url, headers, local_filename)
More
Howto was created based on system version 1.27.0.0 (05/2024) and presents functions that may not be available on your system. Ask AMAGE about providing this functionality. |
Due to ongoing development of the system, some screens or configuration files may look slightly different, but will still retain the full functionality described here. This does not affect the core functionality described in this document. |